Code Snippet: How to upload an image with FTPS in ASP.NET Core
by George Kosmidis / Published 5 years ago
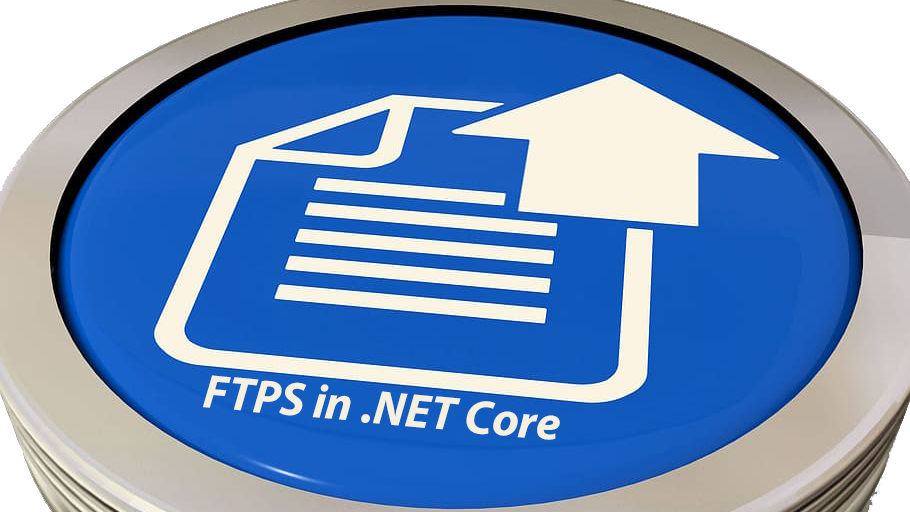
This post is contains sample on how to upload an image with FTPS in ASP.NET Core. It also contains a cheat sheet of all the properties and methods of an instance of an FtpWebRequest
object, mostly as a quick reference guide.
The sample!
I know it is usually the other way around, but I kind of feel this is the most important part of this post! If you want to learn more about all the properties and methods of an instance of FtpWebRequest
, keep scrolling!
public class SampleFTP {
//...
public async Task Upload(IFormFile file)
{
//Create an FtpWebRequest
var request = (FtpWebRequest)WebRequest.Create("ftp://your_ftp_server/and_path/" + file.FileName);
//Set the method to UploadFile
request.Method = WebRequestMethods.Ftp.UploadFile;
//Set the NetworkCredentials
request.Credentials = new NetworkCredential("your_username", "your_password");
//Set buffer length to any value you find appropriate for your use case
byte[] buffer = new byte[1024];
var stream = file.OpenReadStream();
byte[] fileContents;
//Copy everything to the 'fileContents' byte array
using (var ms = new MemoryStream())
{
int read;
while ((read = stream.Read(buffer, 0, buffer.Length)) > 0)
{
ms.Write(buffer, 0, read);
}
fileContents = ms.ToArray();
}
//Upload the 'fileContents' byte array
using (Stream requestStream = request.GetRequestStream())
{
requestStream.Write(fileContents, 0, fileContents.Length);
}
//Get the response
// Some proper handling is needed
var response = (FtpWebResponse) request.GetResponse();
return response.StatusCode == FtpStatusCode.FileActionOK;
}
//...
}
Properties of an instance of an FtpWebRequest
object.
The following is a table containing all properties of an instance of an FtpWebRequest
object. In bold you will find the ones mostly used and in italics the properties not supported.
Property | Short explanation |
---|---|
string Method { get; set; } | Gets or sets the command to send to the FTP server. (e.g. request.Method = WebRequestMethods.Ftp.UploadFile; )Check the container class of the System.Net.WebRequestMethods.Ftp class for available commands. |
ICredentials Credentials { get; set; } | Set the credentials used to communicate with the FTP server. (e.g. request.Credentials = new NetworkCredential(username, password); ) |
bool KeepAlive { get; set; } | Keep the connection alive after the request completes. Default is true. (e.g. request.KeepAlive = true; ) |
bool UsePassive { get; set; } | Client should initiate a connection on the data port. Default is true. (e.g. request.UsePassive = true; ) |
bool EnableSsl { get; set; } | Set control and data transmissions to be encrypted. Default is true. (e.g. request.EnableSsl= true; ) |
bool UseBinary | Indicate to the server that the data to be transferred is binary. Default is true. (e.g. request.UseBinary = true; ) |
int Timeout { get; set; } | Set the number of milliseconds to wait for a request. (e.g. request.Timeout = 5000; ) |
string RenameTo { get; set; } | Set the new name of a file being renamed. (e.g. request.RenameTo = "new_name"; ) |
AuthenticationLevel AuthenticationLevel { get; set; } | Indicate the level of authentication and impersonation used for this request. (e.g. request.AuthenticationLevel = System.Net.Security.AuthenticationLevel.MutualAuthRequested; ) |
X509CertificateCollection ClientCertificates { get; set; } | Set the certificates used for establishing an encrypted connection to the FTP server. (e.g. request.ClientCertificates = new System.Security.Cryptography.X509Certificates.X509CertificateCollection(); ) |
string ConnectionGroupName { get; set; } | Set the name of the connection group that contains the service point used to send the current request. (e.g. request.ConnectionGroupName = "The .NET Lab"; ) |
long ContentOffset { get; set; } | Byte offset into the file being downloaded by this request. (e.g. request.ContentOffset = 512; ) |
IWebProxy Proxy { get; set; } | Set the proxy used to communicate with the FTP server. (e.g. request.Proxy = new WebProxy(); ) |
int ReadWriteTimeout { get; set; } | Set a time-out when reading from or writing to a stream. (e.g. request.ReadWriteTimeout = 30; ) |
Uri RequestUri { get; } | Get the URI requested by this instance. (e.g. var uri = request.RequestUri; ) |
ServicePoint ServicePoint { get; } | Get the System.Net.ServicePoint object used to connect to the FTP server.(e.g. var servicepoint = request.ServicePoint; ) |
WebHeaderCollection Headers { get; set; } | It’s always empty! |
bool PreAuthenticate { get; set; } | Not supported! |
string ContentType { get; set; } | Not supported! |
long ContentLength { get; set; } | Value is ignored, don’t use it! |
bool UseDefaultCredentials { get; set; } | Not supported! |
Methods of an instance of an FtpWebRequest
object.
Same as before, the most common methods can be found with bold and in the top!
Property | Short explanation |
---|---|
.Create() | Initialize a new System.Net.WebRequest instance for the specified URI scheme.(e.g. var request = (FtpWebRequest)WebRequest.Create(host + "/" + path + "/" + filename); ) |
WebResponse GetResponse(); | Retrieve the stream used to upload data to an FTP server. (e.g. WebResponse response = request.GetResponse(); ) |
Task<WebResponse> GetResponseAsync(); | Retrieve the stream used to upload data to an FTP server asynchronously. (e.g. var response = await request.GetResponse(); ) |
void Abort(); | Terminate an asynchronous FTP operation. (e.g. request.Abort(); ) |
IAsyncResult BeginGetRequestStream(AsyncCallback callback, object state); | Begin asynchronously opening a request’s content stream for writing. (e.g. request.BeginGetRequestStream(new AsyncCallback(GetRequestStreamCallback), request); )Check the Microsoft Docs for more info. |
Stream EndGetRequestStream(IAsyncResult asyncResult); | Begin asynchronously opening a request’s content stream for writing. (e.g. Stream postStream = request.EndGetRequestStream(asynchronousResult); )Check the Microsoft Docs for more info. |
IAsyncResult BeginGetResponse(AsyncCallback callback, object state) | Begin sending a request and receiving a response from an FTP server asynchronously. (e.g. IAsyncResult result= )Check the Microsoft Docs for more info. |
WebResponse EndGetResponse(IAsyncResult asyncResult) | Ends a pending asynchronous operation started with BeginGetResponse(System.AsyncCallback,System.Object) .(e.g. myRequestState.response = (HttpWebResponse) myHttpWebRequest2.EndGetResponse(asynchronousResult); )Check the Microsoft Docs for more info. |
Stream GetRequestStream(); | Retrieve the stream used to upload data to an FTP server. (e.g. var stream = request.GetRequestStream(); )Check the Microsoft Docs for more info. |
Task<Stream> GetRequestStreamAsync(); | Retrieve the stream used to upload data to an FTP server asynchronously. (e.g. var stream = await request.GetRequestStreamAsync(); ) |
object InitializeLifetimeService(); | Obtain a lifetime service object to control the lifetime policy for this instance. (e.g. var service = request.InitializeLifetimeService(); ) |
object GetLifetimeService(); | Retrieve the current lifetime service object that controls the lifetime policy for this instance. (e.g. var service = request.GetLifetimeService(); ) |